Subscribe to my youtube channel for Video Tutorials: https://www.youtube.com/@LibraryofCelsus
(Channel not launched yet)
For an example of how this is used, see:
https://github.com/libraryofcelsus/Basic-Oobabooga-Chatbot
https://github.com/libraryofcelsus/Basic-OpenAi-Chatbot
Step 1: Setting Up the Conversation Class #
The first step is to define the core of our system: a class to manage the conversation. We’ll name it MainConversation.
- Our class will need to store settings like the maximum number of entries in the history (max_entries), a main system prompt, and a greeting message.
import os
import json
class MainConversation:
def __init__(self, max_entries, prompt, greeting):
try:
# Set Maximum conversation Length
self.max_entries = max_entries
# Set path for Conversation History
self.file_path = f'./main_conversation_history.json'
# Set Main Conversatoin with Main and Greeting Prompt
self.main_conversation = [prompt, greeting]
2. If a history file exists, we will load it. Otherwise, we’ll start with an empty conversation list to avoid errors.
if os.path.exists(self.file_path):
with open(self.file_path, 'r', encoding='utf-8') as f:
data = json.load(f)
self.running_conversation = data.get('running_conversation', [])
else:
self.running_conversation = []
Step 2: Appending to the Conversation #
We’ll add a method to our class that allows us to append new exchanges to the history.
- Define an upper-case username and chatbot name, upper-case is used as most LLM models use this format.
def append(self, user_input, output):
usernameupper = EXAMPLE_USERNAME
botnameupper = EXAMPLE_BOTNAME
2. This involves creating an entry for our exchange and appending it to our conversation list. Each entry is then separated by a newline for model formatting.
entry = []
entry.append(f"{usernameupper}: {user_input}")
entry.append(f"{botnameupper}: {output}")
self.running_conversation.append("\n".join(entry))
3. If our history becomes too long, we’ll remove the oldest entries. To do this we will first read the number of items with len and then use pop(0) to remove the first entry. Once the first entry has been removed, we will save the list to the json.
while len(self.running_conversation) > self.max_entries:
self.running_conversation.pop(0)
self.save_to_file()
Step 3: Saving the Conversation to a File #
To persist the conversation history, we will save it to a JSON file.
- First we will need to create a variable containing the main conversation and the running conversation.
def save_to_file(self):
# Combine main conversation and formatted running conversation for saving to file
data_to_save = {
'main_conversation': self.main_conversation,
'running_conversation': self.running_conversation
}
2. Then the joined list is saved to a json file with utf-8 encoding. Not using utf-8 encoding will lead to errors if special characters are generated in a reply.
# Save the joined list to a json file
with open(self.file_path, 'w', encoding='utf-8') as f:
json.dump(data_to_save, f, indent=4)
Step 4: Retrieving the Conversation History #
When needed, we can retrieve the entire history.
- The last function we need to create is a function for returning the conversation history when it is called. First we will check for an existing conversation, if none exists, it will create a conversation list with the main and greeting prompts.
def get_conversation_history(self):
if not os.path.exists(self.file_path):
self.save_to_file()
2. After the conversation history has been loaded, we then need to split each entry and add a linebreak between them to maintain formatting.
def get_conversation_history(self):
if not os.path.exists(self.file_path):
self.save_to_file()
# Join Main Conversation and Running Conversation
return self.main_conversation + ["\n".join(entry.split(" ")) for entry in self.running_conversation]
Step 5: Implementing the Conversation History #
With the class defined, we can now use it:
- First we need to define the needed variables and pass them through to the conversation class.
main_prompt = "EXAMPLE MAIN SYSTEM PROMPT"
greeting_prompt = "EXAMPLE GREETING"
max_entries = 12
main_conversation = MainConversation(max_entries, main_prompt, greeting_prompt)
2. Next we will get the conversation history from the json file using the previously defined get_conversation_history function.
conversation_history = main_conversation.get_conversation_history()
3. The final step will be to update the list with the user input and bot response.
# Append json file with newest response
main_conversation.append(user_input, output)
4. Now pass through the conversation_history variable as your conversation history list!
conversation.append({'content': f"{conversation_history}"})
With that you now have a custom conversation history list! #
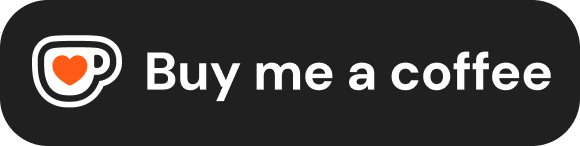
License
MIT License
Copyright (c) 2023 LibraryofCelsus.com
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the “Software”), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED “AS IS”, WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE.